Excel File Uploads in Laravel: How to Solve Common Errors and Ensure Data Quality
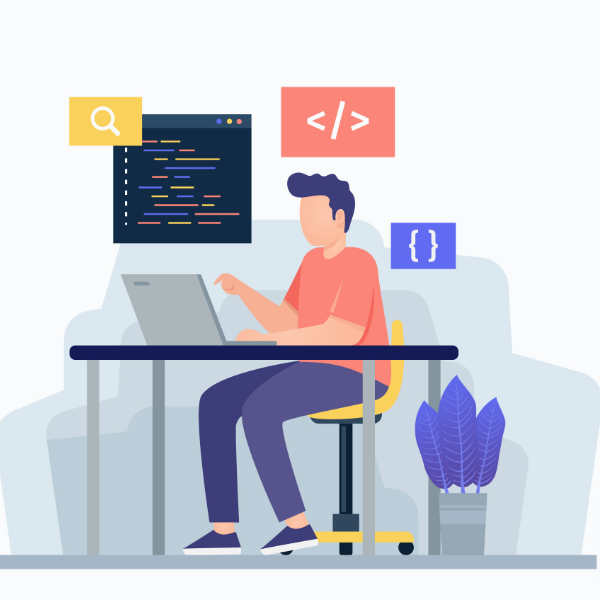
Excel File Uploads in Laravel: How to Solve Common Errors and Ensure Data Quality
January 12, 2023
Excel file uploads can be a convenient way to manage data, but they can also be a source of frustration when errors occur. In this blog post, we will discuss the top 5 common issues that can occur when uploading Excel files in Laravel, and how to solve them.
- Incorrect data types: One of the most common issues when uploading Excel files is that the data is not in the correct format. For example, a date may be entered as text, causing errors when trying to upload the file. To solve this issue in Laravel, you can use the following code to check the data types of each cell and convert them to the correct format before uploading:
use Illuminate\Support\Facades\Validator;
use Illuminate\Support\Facades\Input;
$validator = Validator::make(Input::all(), [
'file' => 'required|mimes:xlsx,csv',
]);
This code uses Laravel’s built-in validation to ensure that the file being uploaded is in the correct format (either .xlsx or .csv). If the file does not meet this requirement, the validation will fail and an error message will be returned to the user.
- Missing data: Another common issue when uploading Excel files is missing data in required fields. This can cause errors when trying to upload the file. To solve this issue in Laravel, you can use the following code to check for missing data before uploading:
use Illuminate\Support\Facades\Validator;
use Illuminate\Support\Facades\Input;
$validator = Validator::make(Input::all(), [
'file' => 'required|mimes:xlsx,csv',
'column1' => 'numeric',
'column2 => 'date'
]);
This code uses Laravel’s built-in validation to ensure that the required columns (in this example, column1 and column2) are not empty. If any of the required columns are empty, the validation will fail and an error message will be returned to the user.
- Inconsistent data format: Another common issue when uploading Excel files is an inconsistent data format. For example, some cells may be formatted as text while others are numbers. To solve this issue in Laravel, you can use the following code to check for inconsistent data format before uploading:
use Illuminate\Support\Facades\Validator;
use Illuminate\Support\Facades\Input;
$validator = Validator::make(Input::all(), [
'file' => 'required|mimes:xlsx,csv',
'column1' => 'required',
'column2 => 'required'
]);
This code uses Laravel’s built-in validation to ensure that the data in the specified columns (in this example, column1 and column2) is in the correct format (numeric and date, respectively). If the data in any of the columns is not in the correct format, the validation will fail and an error message will be returned to the user.
- Extra spaces or characters: Another common issue when uploading Excel files is extra spaces or characters in the data. This can cause errors when trying to upload the file. To solve this issue in Laravel, you can use the following code to check for extra spaces or characters before uploading:
use Illuminate\Support\Facades\Validator;
use Illuminate\Support\Facades\Input;
$validator = Validator::make(Input::all(), [
'file' => 'required|mimes:xlsx,csv',
'column1' => 'string|regex:/^[\S]+$/',
'column2' => 'string|regex:/^[\S]+$/'
]);
This code uses Laravel’s built-in validation to ensure that the specified columns (in this example, column1 and column2) contain only non-whitespace characters. The ‘regex’ validation rule is used to check if there are any extra spaces or characters in the data. If any extra spaces or characters are found, the validation will fail and an error message will be returned to the user.
- Large numbers or long decimals: Another common issue when uploading Excel files is large numbers or long decimals. This can cause errors when trying to upload the file. To solve this issue in Laravel, you can use the following code to check for large numbers or long decimals before uploading:
use Illuminate\Support\Facades\Validator;
use Illuminate\Support\Facades\Input;
$validator = Validator::make(Input::all(), [
'file' => 'required|mimes:xlsx,csv',
'column1' => 'numeric|digits_between:1,5',
'column2' => 'numeric|digits_between:1,5',
'column3' => 'numeric|max:9999999999.99'
]);
This code uses Laravel’s built-in validation to ensure that the specified columns (in this example, column1, column2 and column3) contain only numbers that are within the specified range of digits and decimal places. The ‘digits_between’ and ‘max’ validation rules are used to check if the numbers are within the allowed range. If any numbers are found to be out of range, the validation will fail and an error message will be returned to the user.
In conclusion, Excel file uploads can be a powerful tool for managing data, but they can also be a source of frustration when errors occur. By using the techniques discussed in this blog post, you can use Laravel’s built-in validation to catch and solve common issues that may arise when uploading Excel files. These include incorrect data types, missing data, inconsistent data format, extra spaces or characters, and large numbers or long decimals. By addressing these issues before uploading, you can ensure that your data is accurate and error-free, making it easier to work with and analyze. If you wish to not spend time and effort reinventing the wheel by writing code to solve above error or similar error then checkout highly recommended solution to use inbuilt upload file functionality using boomxl.com. Feel free to reach us out if you need help from our “Product Expert”
Contact
Get Connected Now!
Got a question? We are here to help!
Where to Find Us
222 Broadway, 19th Floor,
New York, NY 10038, USA