Laravel Excel Uploads: Mastering the Challenges of Data Onboarding and Building a Robust Functionality
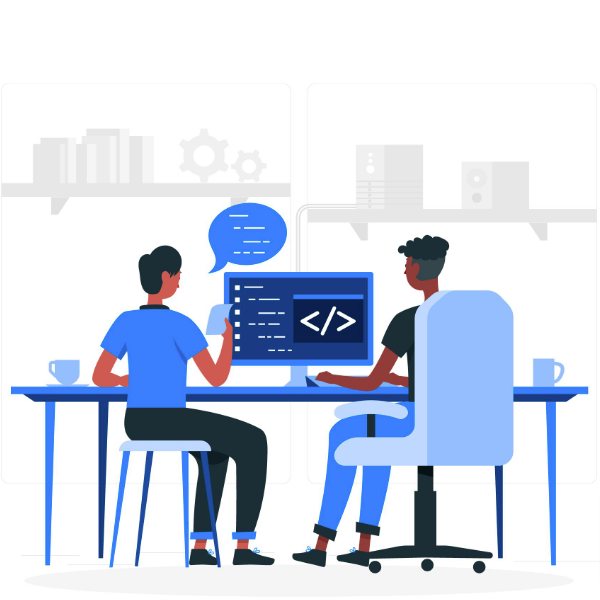
Laravel Excel Uploads: Mastering the Challenges of Data Onboarding and Building a Robust Functionality
March 11, 2019
Excel file uploads are a commonly used feature in many web applications, particularly for data onboarding into a product. However, building a robust and reliable excel upload functionality in Laravel can be challenging. In this blog post, we will discuss the process of building an excel upload functionality in Laravel and the challenges that may arise during this process.
First, let’s start with the basic setup of the excel upload functionality in Laravel. To begin, we will need to install a package called “phpoffice/phpspreadsheet” which provides a set of classes for working with spreadsheet files. To install it, run the following command in your terminal:
composer require phpoffice/phpspreadsheet
Once the package is installed, we can start building the excel upload functionality. In the controller, we will create a function that handles the file upload and processes the data.
The following is a sample code for handling the file upload:
use Illuminate\Http\Request;
use PhpOffice\PhpSpreadsheet\IOFactory;
class ExcelController extends Controller
{
public function upload(Request $request)
{
$request->validate([
'file' => 'required|mimes:xlsx,csv'
]);
$file = $request->file('file');
$spreadsheet = IOFactory::load($file);
$data = $spreadsheet->getActiveSheet()->toArray();
// Process data
}
}
The above code first validates that a file was indeed uploaded and that it is in the correct format (either .xlsx or .csv). Then it loads the file into a “spreadsheet” object which can be used to access the data in the file. The data is then converted into an array and can be processed as needed
Now that we have the basic excel upload functionality set up, let’s discuss some of the challenges that may arise during this process. Handling large files: One of the biggest challenges when building an excel upload functionality is handling large files. These files can take a long time to process and may cause performance issues in the application. One way to solve this issue is to use a package like “maatwebsite/excel” which provides a more efficient way to handle large files.
use Maatwebsite\Excel\Facades\Excel;
class ExcelController extends Controller
{
public function upload(Request $request)
{
$request->validate([
'file' => 'required|mimes:xlsx,csv'
]);
Excel::filter('chunk')->load($request->file('file'))->chunk(250, function($results){
// Process data
});
}
}
The above code uses the ‘maatwebsite/excel’ package to handle large files. The ‘filter’ and ‘chunk’ methods are used to process the file in chunks, making it more efficient for handling large files.
Data validation: Another challenge when building an excel upload functionality is validating the data in the file. This includes checking for missing data, incorrect data types, and inconsistent data format. Laravel provides built-in validation functions that can be used to validate the data before it is processed. However, it’s also important to make sure that the validation rules are specific and robust enough to catch all possible errors.
use Illuminate\Support\Facades\Validator;
use Illuminate\Support\Facades\Input;
class ExcelController extends Controller
{
public function upload(Request $request)
{
$validator = Validator::make(Input::all(), [
'file' => 'required|mimes:xlsx,csv',
'column1' => 'required',
'column2 => 'date'
]);
if($validator->fails()) {
return redirect()->back()->withErrors($validator);
}
$file = $request->file('file');
$spreadsheet = IOFactory::load($file);
$data = $spreadsheet->getActiveSheet()->toArray();
// Process data
}
}
The above code uses Laravel’s built-in validation to check for missing data in the required column and the format of date in column2. If the validation fails, it returns the error message to the user.
Data Integrity: When building an excel upload functionality, it’s important to ensure that the data is accurate and consistent. This may involve checking for duplicate data, enforcing constraints, and ensuring that the data is in the correct format. One way to solve this issue is to use database triggers or foreign keys to enforce these constraints.
use Illuminate\Support\Facades\DB;
class ExcelController extends Controller
{
public function upload(Request $request)
{
$file = $request->file('file');
$spreadsheet = IOFactory::load($file);
$data = $spreadsheet->getActiveSheet()->toArray();
DB::transactions(function() use ($data){
// Process data and insert into database
// Enforce constraints using foreign keys or database triggers
});
}
}
The above code uses Laravel’s built-in database transaction function to ensure that the data is accurate and consistent. It also enforces constraints using foreign keys or database triggers to ensure data integrity.
Error handling: Another challenge when building an excel upload functionality is handling errors that may occur during the process. This includes catching and displaying error messages to the user, as well as logging errors for debugging purposes. Laravel provides built-in error handling functions that can be used to handle these errors in a clean and organized way.
class ExcelController extends Controller
{
public function upload(Request $request)
{
try{
$file = $request->file('file');
$spreadsheet = IOFactory::load($file);
$data = $spreadsheet->getActiveSheet()->toArray();
// Process data
} catch(\Exception $e) {
\Log::error($e->getmessage());
return redirect()->back()->withErrors("An error occoured while processing the file. Please try again");
}
}
}
The above code uses a try-catch block to handle any errors that may occur during the process. It logs the error message for debugging purposes and returns
In conclusion, building an excel upload functionality in Laravel can be challenging, but with the right tools and techniques, it’s a powerful way to manage and onboard data into a product. One possible solution to this challenge is to use a service like boomxl.com, which provides a pre-built excel upload functionality that can be easily integrated into your Laravel application. This allows you to bypass the need to build the functionality from scratch and focus on customizing it to fit your specific needs.
We have discussed the process of building an excel upload functionality in Laravel, including the basic setup, handling large files, data validation, data integrity, and error handling. By using the techniques discussed in this blog post, you can build a robust and reliable excel upload functionality that can handle large files, validate data, ensure data integrity and handle errors in a clean and organized way.
Furthermore, the challenges that may arise during this process have been discussed, such as handling large files, data validation, data integrity, and error handling. With the right approach and tools, these challenges can be overcome to ensure that the data is accurate, consistent and error-free. Additionally, the use of packages like ‘phpoffice/phpspreadsheet’ and ‘maatwebsite/excel’ and Laravel’s built-in validation, transaction, and error handling functions can make the process of building an excel upload functionality much more efficient.
In summary, excel upload functionality is a key feature in many web applications and building it in Laravel can be challenging, but with the right tools and techniques, it’s a powerful way to manage and onboard data into a product. By addressing and solving the common issues that may arise when uploading Excel files, you can ensure that your data is accurate, consistent, and error-free. Additionally, using a service like boomxl.com can be a great solution for those who want to bypass the need to build the functionality from scratch and focus on out of the box ready to use upload functionality button and still have an option to customizing it to fit their specific needs.
Contact
Get Connected Now!
Got a question? We are here to help!
Where to Find Us
222 Broadway, 19th Floor,
New York, NY 10038, USA